AI-Infused Consulting: Experimenting with Real-Time AWS Insights
Build an AI Consulting Agent that delivers real-time, context-aware technical insights.
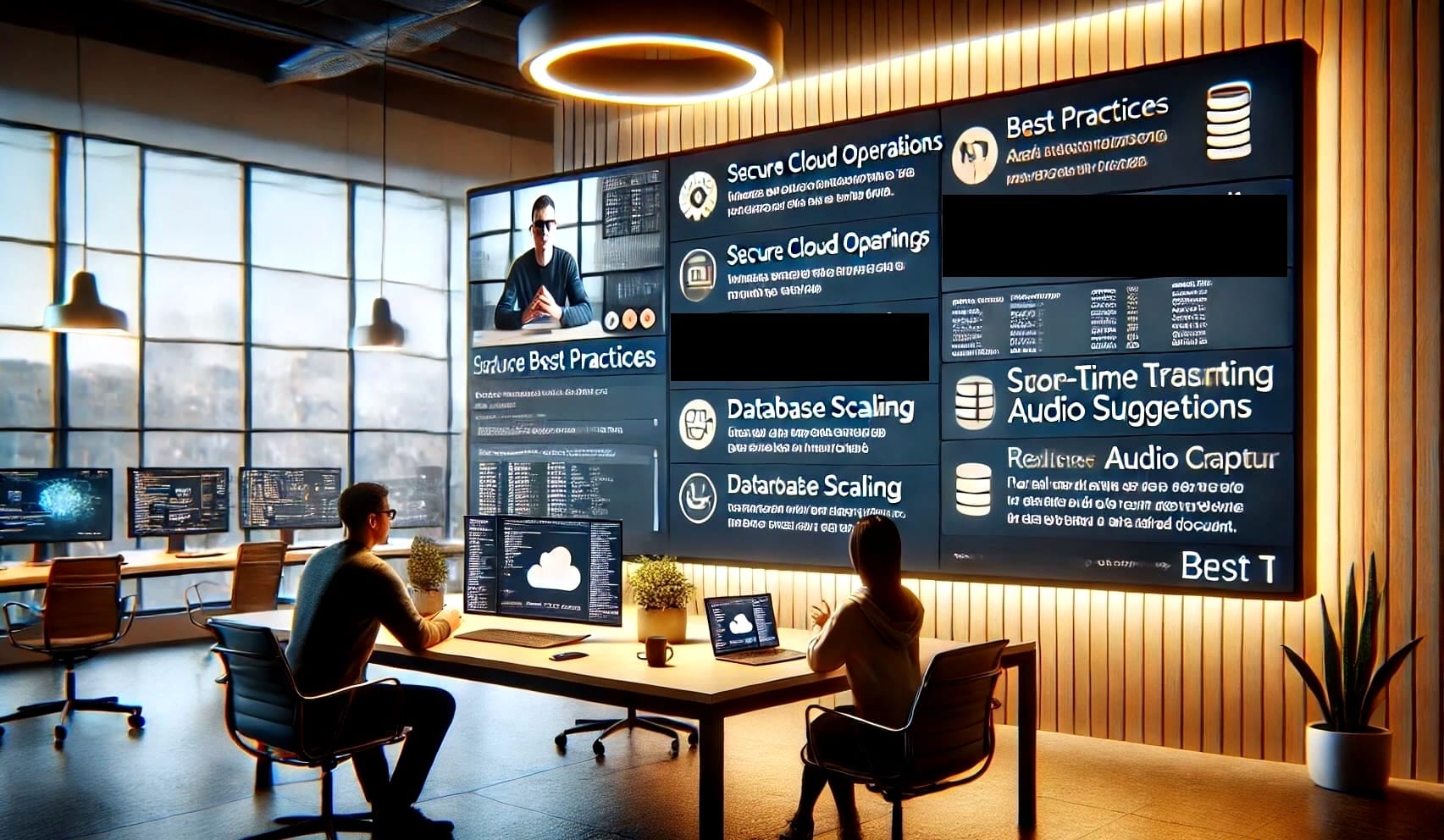
I regularly encounter people asking for tips on effectively using AWS. Although I’ve been working with AWS since 2009, there are over 200 services, and no single person can possibly master all of them. Over the years, I’ve seen customers, partners, and internal AWS teams alike struggling to navigate this expansive ecosystem—from choosing the right compute service to securing applications using DevSecOps best practices. This is one of the reasons I keep a collection of blog posts, documentation links, and reference notes. This habit isn’t about lacking knowledge; rather, it’s a way to verify information in real time and deliver the most accurate insights.
Yet, every time I jump into a detailed architecture or software delivery discussion, I feel the drag of scouring multiple sources for references. That’s when I started thinking about an AI-enabled assistant that not only surfaces the right documentation but also follows conversations, understands context, and delivers real-time suggestions. Think about being in the middle of an AWS architecture discussion, and the moment you mention “Amazon DynamoDB scaling,” an AI agent shows curated best practices from official AWS docs. In essence, you have a consultant for the consultant: an AI Consulting Agent.
Offloading repetitive research tasks to AI lets you focus on bigger-picture issues. Instead of switching between a half-dozen browser tabs, you let a smart system listen to your conversation, offering timely, context-specific references and solutions.
Application Architecture
Application Architecture
The application I've been developing acts as an AI Consulting Agent. It offers real-time transcription, LLM-based technical recommendations, and automated documentation updates. The examples use Google Cloud Speech-to-Text for transcription. You can easily swap it for Amazon Transcribe if you want to stay within AWS. Here are the main components:
- Meeting Audio Capture
- Platform Integration:
Currently, the application supports Google Meet for direct integrations and local recordings for flexible audio capture. - Audio Retrieval Methods:
- Google Meet: Leverages API/webhook integrations to capture live audio streams during a meeting.
- Local Recordings: Records audio locally and feeds it into the transcription service, enabling you to work with pre-recorded content.
- Platform Integration:
- Transcription & Recommendation
- Transcription Options:
- Google Cloud Speech-to-Text: The app uses Google’s transcription service by authenticating with OAuth credentials and creating a
SpeechClient
for near-real-time audio streaming. - Amazon Transcribe: For those who prefer an AWS-centric approach, the integration can be easily adapted to use Amazon Transcribe, which offers benefits such as built-in PII redaction, deep S3 integration, and support for long-duration streaming (up to 4 hours).
- Google Cloud Speech-to-Text: The app uses Google’s transcription service by authenticating with OAuth credentials and creating a
- LLM Recommendations:
Once the audio is transcribed, the transcript is forwarded to an LLM (such as GPT-4) via an AWS Lambda function. This Lambda function:- Receives the transcript.
- Calls the LLM to generate detailed technical recommendations.
- Implements exponential backoff to handle rate-limit errors gracefully.
- Transcription Options:
- Documentation Update
- Google Docs Integration:
After generating recommendations, the application automatically appends the structured guidance to a shared Google Doc. - Authentication & Token Management:
The Google Docs service is created using OAuth credentials securely stored in AWS Systems Manager (SSM), ensuring that tokens are refreshed automatically when needed.
- Google Docs Integration:
Core Code Snippets
Below are three code snippets demonstrating how to integrate Google Docs, GPT-4, and Google Cloud Speech-to-Text. (You can adapt these to Amazon Transcribe and other LLMs with minimal changes.)
Snippet 1: Creating the Google Docs Service
When your AI agent adds new content to a Google Doc, it must be authorized. This code checks for missing scopes, refreshes tokens, and updates AWS Systems Manager (SSM) if credentials are expired.
import json
import boto3
from google.auth.transport.requests import Request
from google.oauth2.credentials import Credentials
from googleapiclient.discovery import build
def create_docs_service(creds_data):
"""Create and return a Google Docs service."""
required_scopes = GOOGLE_DOCS_REQUIRED_SCOPES
current_scopes = set(creds_data.get('scopes', []))
missing_scopes = required_scopes - current_scopes
if missing_scopes:
creds_data['scopes'] = list(current_scopes | missing_scopes)
credentials = Credentials(
token=creds_data['token'],
refresh_token=creds_data['refresh_token'],
token_uri=creds_data['token_uri'],
client_id=creds_data['client_id'],
client_secret=creds_data['client_secret'],
scopes=creds_data['scopes']
)
if credentials.expired or missing_scopes:
credentials.refresh(Request())
creds_data['token'] = credentials.token
ssm = boto3.client('ssm')
ssm.put_parameter(
Name=SSM_PARAMS['GOOGLE_OAUTH_TOKENS'],
Value=json.dumps(creds_data),
Type='SecureString',
Overwrite=True
)
print("Updated token saved to SSM")
return build('docs', 'v1', credentials=credentials)
Key Points
- Pulls OAuth data from SSM.
- Ensures your credential scopes match what Google Docs requires.
- Automatically refreshes tokens if they’re expired.
- Returns a docs service object you can call to edit a Google Doc programmatically.
Snippet 2: Generating Recommendations via GPT-4
After the AI agent receives a transcript, it calls GPT-4 to produce structured technical guidance. Note the exponential backoff in case of rate-limit errors:
import time
import random
from openai import OpenAIError
def get_openai_recommendation(transcript, client):
"""Get recommendations from OpenAI based on the transcript."""
messages = [
{
"role": "system",
"content": (
"You are a Senior Principal Solutions Architect...\n"
"CRITICAL INSTRUCTIONS:\n"
"1. Provide ONLY specific technical solutions.\n"
"2. ...\n"
"3. Include concrete details...\n"
)
},
{
"role": "user",
"content": f"Here is the meeting transcript:\n{transcript}"
}
]
for attempt in range(5):
try:
response = client.chat.completions.create(
model="gpt-4",
messages=messages,
temperature=0.7,
max_tokens=500
)
return response.choices[0].message.content
except OpenAIError as e:
if "rate_limit" in str(e):
delay = (2 ** attempt) + (random.random() * 0.5)
print(f"Rate limited. Retrying in {delay:.2f}s...")
time.sleep(delay)
else:
raise
raise Exception("Failed after 5 retries")
Highlights
- The system instructions ensure GPT-4 yields domain-specific, direct answers (e.g., recommended AWS services, config details).
- If rate-limited by OpenAI, the code sleeps and retries to avoid a total failure.
- The final text can then be logged in a Google Doc or similar repository.
Snippet 3: Creating the Google Cloud Speech Client
Your AI agent also needs to authenticate with Google Cloud Speech-to-Text (though you could swap in Amazon Transcribe). This snippet shows how to manage OAuth tokens:
import json
from google.auth.transport.requests import Request
from google.oauth2.credentials import Credentials
from google.cloud import speech
def create_speech_client(creds_data):
"""Create and return a Google Cloud Speech-to-Text client."""
required_scopes = [
'https://www.googleapis.com/auth/cloud-platform',
'https://www.googleapis.com/auth/cloud-speech'
]
credentials = Credentials.from_authorized_user_info(
info={
'token': creds_data['token'],
'refresh_token': creds_data['refresh_token'],
'token_uri': creds_data['token_uri'],
'client_id': creds_data['client_id'],
'client_secret': creds_data['client_secret'],
'scopes': required_scopes
},
scopes=required_scopes
)
if credentials.expired and credentials.refresh_token:
credentials.refresh(Request())
creds_data['token'] = credentials.token
# Optionally store updated creds in SSM
return speech.SpeechClient(credentials=credentials), credentials
Key Details
- Pulls Google OAuth credentials from SSM.
- Refreshes tokens when needed.
- Returns a SpeechClient for near-real-time audio streaming from your chosen meeting platform.
Why I Chose Google Cloud Speech-to-Text
You may ask why I’m using Google Cloud Speech-to-Text instead of Amazon Transcribe in a system that otherwise relies on AWS (Lambda, SSM, etc.). Early on, I assumed Google’s solution was significantly cheaper due to free credits offered on new Google Cloud accounts (60 minutes/month plus $300 promotional credit)[1]. However, the reality is that Amazon Transcribe’s rate ($0.024/min for standard English) is comparable to Google’s base rate ($0.024/min for standard models)[2][3][4]. Both providers offer free tiers, volume discounts, and domain-specific enhancements.
Ultimately, the biggest factor for me was ease of integration with my existing Google Account. I found the OAuth model simpler to manage for a real-time streaming scenario. Your mileage may vary. If you want everything under AWS for operational or compliance reasons, Amazon Transcribe is a great choice—especially with built-in PII redaction, deep S3 integration, and the ability to stream up to 4 hours at a time[2][5][7].
Ways to Extend the AI Consulting Agent
1. Retrieval-Augmented Generation (RAG) for Knowledge Bases
Why RAG? Large language models can hallucinate or provide outdated answers if they rely on static training data. Retrieval-Augmented Generation retrieves relevant documentation from an external knowledge base at query time, improving accuracy.
Implementation: You might store your data in Amazon Kendra, Pinecone, or an open-source vector DB like Milvus. When the agent processes a transcript snippet, it queries your knowledge base for relevant content and appends it to the prompt before calling GPT-4 (or another LLM).
2. Amazon Bedrock for Alternative Models
What is Bedrock? A fully managed service that gives you access to multiple foundation models (from AI21, Anthropic Claude, Stability AI, and more) without having to manage AI infrastructure yourself.
Why Use It? GPT-4 is powerful but not the only game in town. Bedrock may offer better latency, security, or cost advantages for certain workloads. For example, Claude might excel at summarization, or Jurassic-2 might handle code generation effectively.
Integration:
- Your AWS Lambda function transcribes the audio.
- It queries the appropriate foundation model via Bedrock.
- The result is appended to your Google Doc (or any preferred output).
3. Multi-Platform Meeting Support: Zoom, Teams, Webex
Although I've configured the application to record locally for multiple platforms, it currently automates Google Meet creation out of the box. Many of your customers, however, may rely on Zoom, Microsoft Teams, or Webex. Supporting these platforms can broaden your solution’s reach.
Key Steps:
- Platform APIs/Webhooks: Retrieve real-time audio or recordings from each platform’s API or webhook.
- Transcription: Forward the audio to your transcription service (Google STT or Amazon Transcribe).
- AI Recommendations: Feed the resulting transcript into your LLM-based recommendation engine.
For example:
- Microsoft Teams: Integrate with Microsoft Graph APIs to schedule/join calls or store transcripts in SharePoint or a Teams channel.
- Zoom: Use Zoom’s Cloud Recording or Streaming APIs to capture audio and process it in near real time.
4. Real-Time Updates Beyond Google Docs (e.g., Slack)
Not everyone wants to check a Google Doc mid-meeting. You could push your AI Consulting Agent’s insights to Slack, Microsoft Teams, or even a custom web UI.
- Slack Integration:
- Create a Slack app with appropriate scopes (e.g., chat:write).
- When GPT-4 returns its recommendations, send them to a specific channel or direct message.
- Use Slack’s Block Kit for richer formatting (headers, bullet points, code blocks).
- Microsoft Teams Messages:
- Similar approach: generate a Teams message via Microsoft Graph API.
- Create an adaptive card or a simple text post containing the recommendations and a link to the recorded transcript.
- Custom UI:
- Build a simple web dashboard that shows real-time AI suggestions.
- Store them in a database (e.g., DynamoDB or Firestore) and update the front end via WebSockets or server-sent events.
By delivering these AI-driven recommendations in real time—directly where stakeholders already communicate—you create a more seamless experience than having them open a doc every time.
5. Addressing GPT-4 (or Other LLM) Costs
Token-Based Pricing: GPT-4 usage can be expensive if your transcripts are large. Each chunk of text you send to the model can rack up tokens quickly.
Mitigation:
- Concise Prompts: Only embed the minimal relevant text in your prompts.
- Use Cheaper Models for Routine Tasks: GPT-3.5 or a smaller open-source model might suffice for simpler tasks.
- Batch Transcriptions: Instead of calling GPT-4 after every short snippet, gather 5–10 minutes of conversation before sending it to the model.
- Experiment with Bedrock: Some models on Bedrock may have lower rates or dedicated enterprise pricing.
- Self-Hosting: If you have large volumes, consider hosting an open-source LLM on Amazon SageMaker or ECS. Upfront engineering is higher, but you control infrastructure costs.
Why It Matters to You: Enabling the “Do” Stage of the AWS Partner Flywheel
In my AWS Partner Flywheel Growth Accelerator, I describe eight main components: Learn, Do, Measure, Share, Validate, Sell, Iterate, and Operate. The “Do” part is about effectively delivering real solutions to customers. An AI Consulting Agent helps you accelerate and refine that “Do” stage by:
- Accelerated Delivery: No more fumbling for docs or outdated wiki pages. You get in-meeting, real-time guidance.
- Consistent Quality: The agent ensures recommended patterns match the current state of AWS, so you meet or exceed the bar every time.
- Scale Expertise: New or less-experienced team members can rely on the AI’s knowledge, enabling them to ramp up quickly and help deliver solutions.
By streamlining knowledge retrieval, you can free your team to focus on strategy, problem-solving, and forging deeper relationships with customers—a direct boost to how you Do business.
Where to Go from Here
Imagine you never have to pause a meeting to Google a snippet of code, check a best-practices doc, or confirm a configuration detail. That’s the promise of an AI Consulting Agent: it does the drudge work so you can concentrate on delivering real solutions for your customers.
If you’re considering something like this, start small—build a proof of concept that integrates Google Cloud Speech-to-Text or Amazon Transcribe, calls GPT-4 (or your model of choice), and logs the output in a single shared doc. Then iterate by adding Retrieval-Augmented Generation with Amazon Kendra or a vector DB, experimenting with Amazon Bedrock models for cost or performance advantages, and opening your system to multiple meeting platforms. You can even integrate Slack or other channels for immediate notifications. This approach not only saves time but also elevates your business's delivery capabilities, aligning with the “Do” stage of the AWS Partner Flywheel.
References
- Google Cloud Speech-to-Text Pricing.
- Amazon Transcribe Pricing.
- Google Cloud Speech-to-Text Enhanced Models.
- Using Amazon Transcribe.
- Comparison of Speech-to-Text APIs.
- Automatic Speech Recognition—AWS Transcribe vs Google STT.
- Hacker News Discussion—AWS Transcribe vs. Google STT.
- Gladia Blog—OpenAI Whisper vs Google STT vs Amazon Transcribe.